7 power features of the Linux shell you really need to know
Chain commands together, use wildcards, and more
The Linux shell is a powerful tool with many features that aren’t immediately obvious to the beginner. In this article, we’ll see some of the hidden power features of the Linux shell which will make your Linux shell experience a whole lot easier. From simple shortcuts to more advanced features, try one of these tricks today.
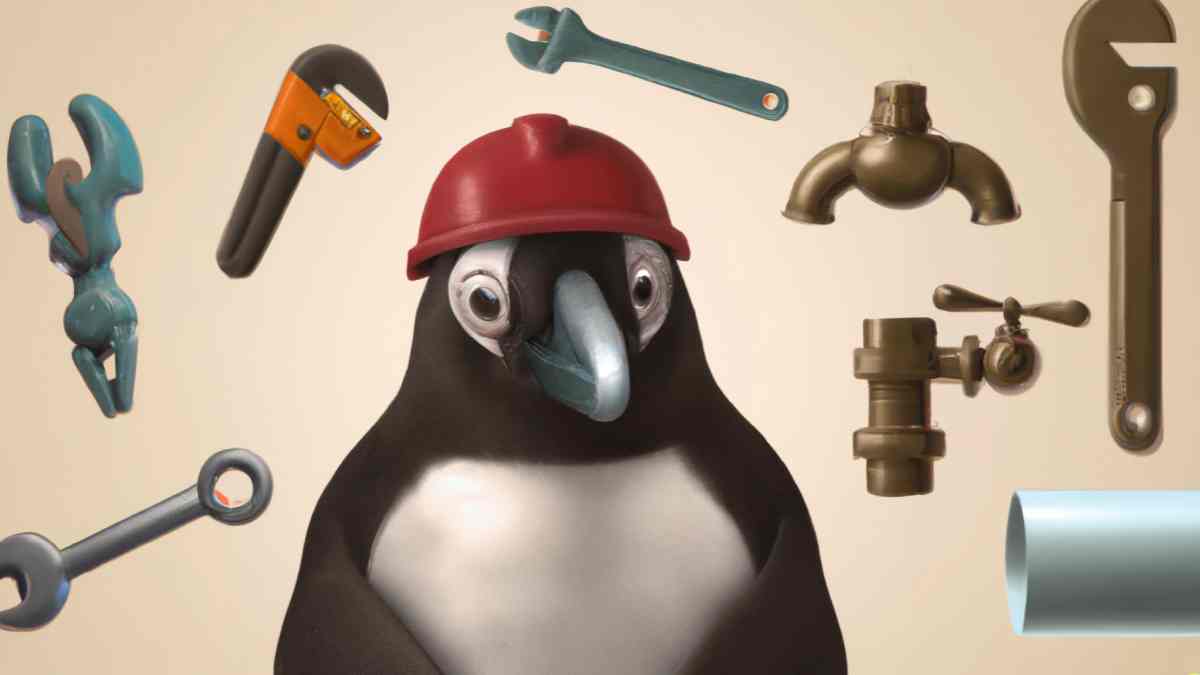
So, I use the Linux shell a lot. I use it for my day job, and I also use it for my personal projects. I use it to do a lot of the legwork for this blog.
I’ve been working with Linux for several years and picked up some useful tips. There are some Linux features which I didn’t know about for years, but now I use them all the time. And there’s still a ton of stuff that I don’t know!
In this post, I want to share some power features which I think are so useful, you’ll want to start using them every day. I’ll also share the proper names of these features, so if you want to know more, you know what to look for in the manual.
And, as a bonus, you’ll sound like you know what you’re talking about at work :-)
These commands work on the Bash or “GNU Bourne-Again SHell” which is the default shell on most Linux distributions. If you’re using a different shell, you might need to use a slightly different command.
Without further ado, let’s get into the seven power features of the Linux shell.
1. Save output from a command to a file
A lot of features in the Linux shell are activated simply by using a special character. So to be a pro, you just need to remember some characters. ;-)
Our first special character in Linux is the humble “greater-than” sign: >
. With this character, you can save the output of a command to a file.
For example, here’s how to use the >
operator to redirect the output of the echo
command, to a file called “hello.txt”:
$ echo "Hello, world!" > hello.txt
This will create a file called hello.txt
and write the text Hello, world!
to it.
This is really useful when you want to share a command’s output with someone. No more do you need to suffer the embarrassment of pulling out your phone and taking a picture of your heavily-stained screen. 🤦♂️ Simply use the >
operator and send them the file! Voila!
Here’s how to use the >
it with another command; let’s use the >
operator to save the output of the ls
command to a file:
$ ls > files.txt
Now you have a file called files.txt
that contains a list of the files in the current directory.
Double up to append to a file >>
You can also use the >>
operator to append to a file. This means that if the file already exists, the output will be added to the end of the file. If the file doesn’t exist, it will be created.
$ echo "Hello, world!" >> hello.txt
This will append the text Hello, world!
to the end of the file hello.txt
.
Run a command and see its output while saving it with ‘tee’
But what if you want to save the output from a command and also see it on the screen? No problem. Just use the tee
command.
Let’s say you want to see the output of the ls
command, but you also want to save the output to a file. You can do this by using the tee
🫖 command:
$ ls | tee files.txt
It’s called “tee” because in plumbing, a “tee” connects two pipes together!
🔵 PROPER NAME IN LINUX: Redirection. To learn more, type man sh
, press /
and search for “redirection”.
2. Run commands in the background
Ever wanted to run a command, but keep using the command line at the same time, and without having to open a new terminal? You can do that with another special character, the ampersand: &
.
With the &
character, you can run a command in the background in a subshell. When you put a command into the background, you can get on with other stuff while the command is doing its thing.
A subshell is a copy of your current shell that runs your background command. You can think of it as a mini-shell that runs your background command and then terminates.
Here’s an example:
$ sleep 1000 &
This example will run the sleep
command in the background for 1,000 seconds, and then exit.
Not sure if your command is still running? You can use the jobs
command to see what’s running in the background:
$ jobs
[1]+ Running sleep 1000 &
Bring a job to the foreground and then to the background again
What if you want to bring a background job into the foreground – so you can see what it’s doing – and then put it back into the background? No problem, this is pretty common! Here’s how it’s done:
-
Run the program: Run your program, adding
&
to run it in the background. -
Bring it to the foreground: Type
fg
, to bring the job back into the foreground. When the job is in the foreground, you’ll see its output in your terminal. -
Suspend the program: Now you can can press
Ctrl+Z
to suspend the command. (During this time, the command isn’t really running, it’s paused. ⏸️) -
Background the program: Finally, type
bg
to put the job back into the background.
Here’s what it looks like:
# start sleep in the background
$ sleep 1000 &
[1] 12345
# bring it to the foreground
$ fg
sleep 1000
# sleep is now running in the foreground, press Ctrl+Z to suspend it
^Z
[1]+ Stopped sleep 1000
$
# sleep is now suspended, type bg to put it back into the background
$ bg
[1]+ sleep 1000 &
🔵 PROPER NAME IN LINUX: Asynchronous commands or background jobs. To learn more, type man sh
, press /
and search for “job control”.
Handy for: Running more than one command at a time. Especially useful if you’re connected to a remote server via SSH and you only have one connection.
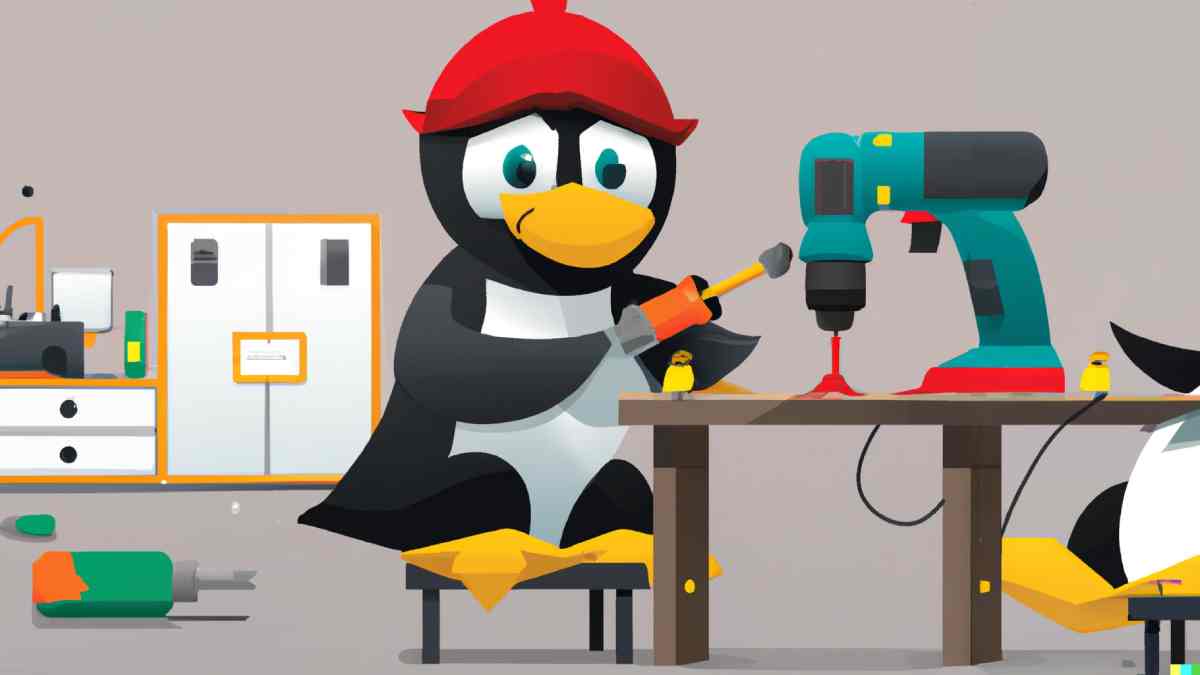
3. Use wildcard patterns to refer to groups of files
You don’t always have to type filenames. There is a handy shortcut!
The shell will automatically expand filenames or lists of files when you use a feature called pattern matching.
Pattern matching lets you find a file or group of files by some pattern in their filename. You can use pattern matching in most of your Linux commands, including ls
, rm
, cp
, mv
, grep
and find
. It’s really helpful because it means you don’t need to type exact filenames all the time!
This feature is really useful when you want to do something to a group of files; like delete them, or move them to a different directory. And don’t we all want to save typing? (Yes, we do.)
To use this feature, just remember the wildcard characters. Here are the most common wildcards:
-
*
matches zero or more characters. (I use this a lot!) -
?
matches exactly one character -
[abc]
matches any one of the charactersa
,b
orc
-
[a-z]
matches any character betweena
andz
(useful for matching all letters of the alphabet!)
For example, you can use the *
wildcard to match any number of characters:
$ ls *.txt
This will print the names of all files that end with .txt
to the screen.
Here’s another example. Let’s print the contents of all files which have four characters in their name and end in .conf
:
$ cat /etc/????.conf
If you ever want to preview what an expansion will look like after it’s been expanded, use the echo
command! For example:
echo cat /etc/????.conf
.
🔵 PROPER NAME IN LINUX: Pathname expansion, pattern matching, “globbing”. To learn more, type man sh
, press /
and search for “pathname expansion”.
What it’s helpful for: Doing something to many files at once; like deleting them, or moving them to a different directory.
4. Pipe output from one command into another
Working in the Linux shell is often about running small commands which do one thing, and then taking the output of that command and passing it as the input to another command.
The pipe operator |
is another special character! It lets you take the output from a command and use it as the input to another command. It’s probably the most useful operator on the Linux command line and I use it every single day.
For example, we can use the ls
command to list the files in a directory, and then pipe the output to the grep
command to search. Here’s an example:
$ ls /etc | grep cron
This example will list the contents of the /etc
directory and then search the output for any files that contain the word cron
in the filename.
Here are some other examples of using the pipe to combine commands:
# Print your command history and search the list for the text 'ls'
$ history | grep ls
# Print all running processes and search the list for the text 'java'
$ ps aux | grep java
# Print the contents of a file and convert double spaces to tabs
$ cat /var/myfile | tr ' ' '\t'
🔵 PROPER NAME IN LINUX: Pipelines. To learn more, type man sh
, press /
and search for “pipelines”.
5. Autocomplete everything
Autocomplete is one of the most useful features of the Linux command line. It’s so useful that I can’t imagine using the command line without it.
Autocomplete is a feature that lets you type the first few letters of a command or filename, and then press Tab
to complete the rest of the word. It’s really useful when you’re typing long filenames or commands.
To start using it, just type a command or a filename.
$ systemd-
Then press Tab
to get a list of possible completions:
$ systemd-
systemd-analyze
systemd-ask-password
systemd-cat
systemd-cgls
...
It’s like the autocomplete feature on your iPhone… except it actually suggests useful stuff.
Some commands have their own autocomplete, too
You can also use autocomplete with some commands!
Yes, many commands have their own autocomplete, too. For example, if you type ls --
and then press the tab key, the command line will show all of the possible options you can use.
And more complex commands, like kubectl (for Kubernetes), have their own autocomplete too. (Check the documentation for the tool you’re using to see if you need to install its completion feature.)
🔵 PROPER NAME IN LINUX: Completion, programmable completion. To learn more, type man bash
, press /
and search for “completion” or “READLINE”.
6. Change text in the command line
How often have you typed a command and realised you’ve got the first part wrong – and needed to go backspace all the way to change it? Or have you ever used a Linux shell and wished you could copy and paste bits of your command?
Well I think this trick is the most hidden of them all. The Linux shell has special key combinations that you can use to cut, copy and paste text in the command line - without needing a mouse:
Move around the command line
You probably know that you can use the arrow keys (Left and Right) to move the cursor around the command line.
But did you know that you can also use these keys too:
-
Ctrl+A
andCtrl+E
keys move to the beginning or end of the line. -
Alt+B
moves the cursor back one word -
Alt+F
moves the cursor forward one word
Cut and edit parts of your command
These key combinations will save you a ton of typing. Use these key combos to edit your typing at the command line:
-
Ctrl-K
will delete everything from the cursor to the end of the line (kind of like deleting forwards) -
Ctrl-U
will delete everything from the cursor to the start of the line (kind of like deleting backwards) -
Ctrl-W
will delete the word before the cursor. -
Ctrl-Y
will paste the last thing you deleted. This is called “yanking” 😳
🔵 PROPER NAME IN LINUX: Killing, yanking (and other weird names). These are features of the “READLINE” part of the shell. To learn more, type man bash
, press /
and search for “READLINE”.
7. Go back in history
When you need to repeat yourself, or run the same command again, you can use the up and down arrow keys to scroll through your command history.
But it gets better. You can also see your command history in the Linux command line. Use the history
command to list the commands that you have run recently:
$ history
Written a long, complicated command and want to run it again? Find the command you want to re-run, and use the !
operator + its number, to run the command again:
$ !123
This will re-run the command that was run in history position 123.
And, most useful of all, you can search for commands in your history too. pressing Ctrl-R will let you search through your history using a text interface. Then just press Enter to run the command again.
Wrapping up
Now you’ve seen a whole bunch of features you probably didn’t even know existed in the Linux command line. Now you’ll be chomping at the bit to get started!
But before you do, there’s one more thing you need to remember…
You can find out more about any command you’re using by using the man
command. For example, to find out more about the ls
command, type:
man ls
I hope you use these hidden features of the Linux shell to make your Linux life easier, and to get things done faster.
Happy Linuxing!