How to download all Maven dependencies into a folder
You can use Maven’s Dependency Plugin to save a copy of all the libraries and JAR files used by your project.
In this article we’ll look at how to use Maven to download all of your Java project’s dependencies, like libraries and frameworks, and save them into a specific folder.
Summary
-
You can use the Maven Dependency Plugin to download dependencies.
-
Run
mvn dependency:copy-dependencies
, to download all your dependencies and save them in thetarget/dependency
folder. -
You can change the target location by setting the property
outputDirectory
.
Normally, you don’t need to download your dependencies explicitly! Maven automatically downloads your project’s dependencies, whenever you run a build. (So you can ignore this post.)
So why might you need to download dependencies? Well, you might need to do this if…
-
You want to run your project (and its dependencies) on another machine which doesn’t have internet access
-
You want to debug a library, so you want a local copy
-
You simply like collecting jars
If this sounds like you, read on.
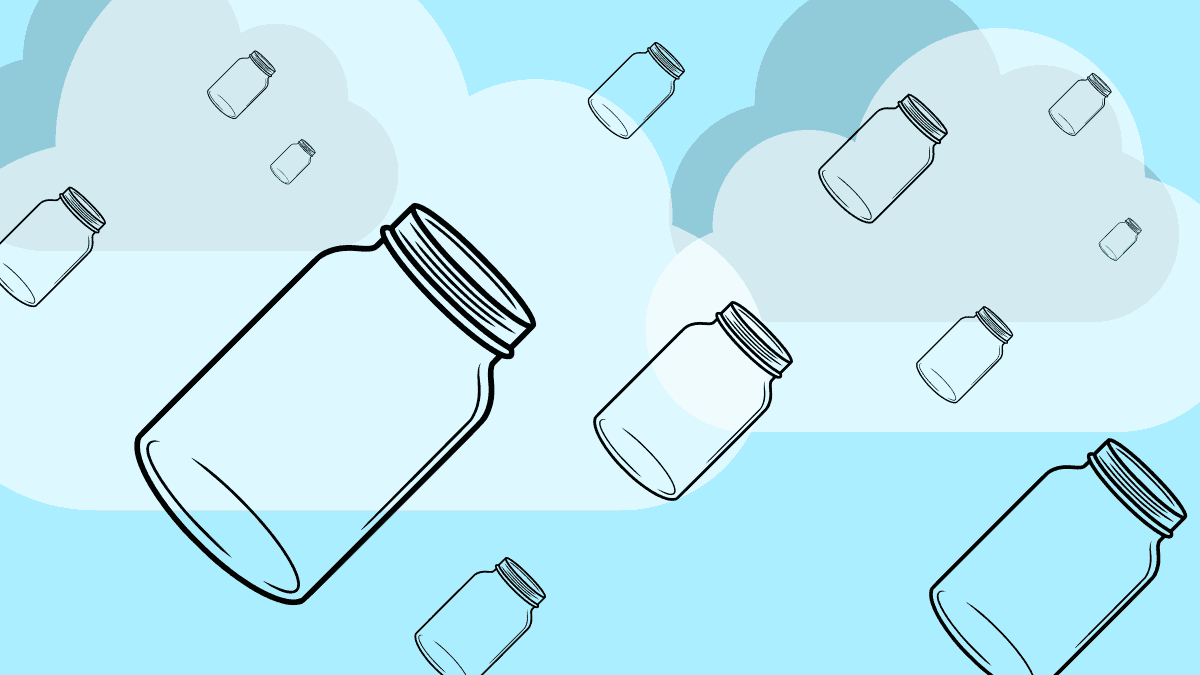
Steps to download all dependencies
When you want to do something in Maven, you usually need a plugin. Most features in Maven – like compiling code, running tests and downloading dependencies – are implemented inside a plugin.
To download dependencies, you can use the Maven Dependency Plugin.
Download dependencies (without building your project)
From the Maven Dependency Plugin, we can use the goal copy-dependencies
. It downloads dependencies from a repository (usually the “main” online repository, Maven Central) to our local project folder.
You can execute this goal separately, without building your project.
Here’s how to do it:
-
In your project’s
pom.xml
, make sure that you’ve added all the dependencies you want to use. -
Drop to a terminal in your project folder (where your
pom.xml
is located). -
Run this command to execute the goal
copy-dependencies
, which starts downloading dependencies:mvn dependency:copy-dependencies
-
Maven Dependency Plugin will download dependencies (for example, JAR files) into the folder
target/dependency
.
Afterwards, you will see that the files have been downloaded:
$ ls -al target/dependency
total 63676
drwxr-xr-x. 2 tdonohue tdonohue 16384 Jun 26 10:46 .
drwxr-xr-x. 7 tdonohue tdonohue 4096 Jun 26 10:46 ..
-rw-r--r--. 1 tdonohue tdonohue 364206 Jun 26 10:46 aesh-2.6.jar
-rw-r--r--. 1 tdonohue tdonohue 6806 Jun 26 10:46 apiguardian-api-1.1.2.jar
-rw-r--r--. 1 tdonohue tdonohue 199943 Jun 26 10:46 arc-2.9.2.Final.jar
-rw-r--r--. 1 tdonohue tdonohue 122176 Jun 26 10:46 asm-9.3.jar
....
And that’s pretty much it.
Download dependencies to a specific location
Want to download to a different location? No problem.
The Maven Dependency Plugin copy-dependency
goal saves files to target/dependency
by default. But you can change the behaviour, by setting the property outputDirectory
.
Here’s an example:
mvn dependency:copy-dependencies -DoutputDirectory=/mydeps
(See how we use -D
to set a property.)
Now Maven will download the artifacts to the /mydeps
directory.
How does it work?
Like most features in Maven, this is made possible by a plugin, the Maven Dependency Plugin. The plugin helps you to manage your project’s dependencies:
About the plugin:
Name | Maven Dependency Plugin |
Purpose | The dependency plugin provides the capability to manipulate artifacts. It can copy and/or unpack artifacts from local or remote repositories to a specified location. |
Homepage | maven-dependency-plugin |
Prefix | dependency: |
Example goals | copy-dependencies – downloads dependenciesanalyze – calculates used/unused dependenciestree – shows the dependency tree for your project |
The plugin analyses the dependencies in your project, and copies dependencies from Maven Central (or the repository you have configured).
There are lots of other plugins for Maven, too - see the list.
Wrapping up
We’ve seen how you can use the Maven Dependency Plugin to download all of your project’s dependencies (JAR files) into a single folder.
We activate this plugin using the dependency:
prefix, and then choose the goal to execute. In our case, we use the copy-dependencies
goal.
If you want to check out what else the plugin can do, see the documentation.
Happy building!